Reactアプリは、互いにネストされた多数のコンポーネントによって形作られています。Reactは、アプリのコンポーネント構造をどのように追跡するのでしょうか?
Reactや他の多くのUIライブラリは、UIをツリーとしてモデル化します。アプリをツリーとして考えることは、コンポーネント間の関係を理解するのに役立ちます。この理解は、パフォーマンスや状態管理などの将来の概念をデバッグするのに役立ちます。
以下を学びます
- Reactがコンポーネント構造を「認識」する方法
- レンダリングツリーとは何か、何に役立つのか
- モジュール依存関係ツリーとは何か、何に役立つのか
UIをツリーとして
ツリーはアイテム間の関係モデルであり、UIはしばしばツリー構造を使用して表現されます。たとえば、ブラウザはツリー構造を使用してHTML(DOM)およびCSS(CSSOM)をモデル化します。モバイルプラットフォームもツリーを使用してビュー階層を表現します。
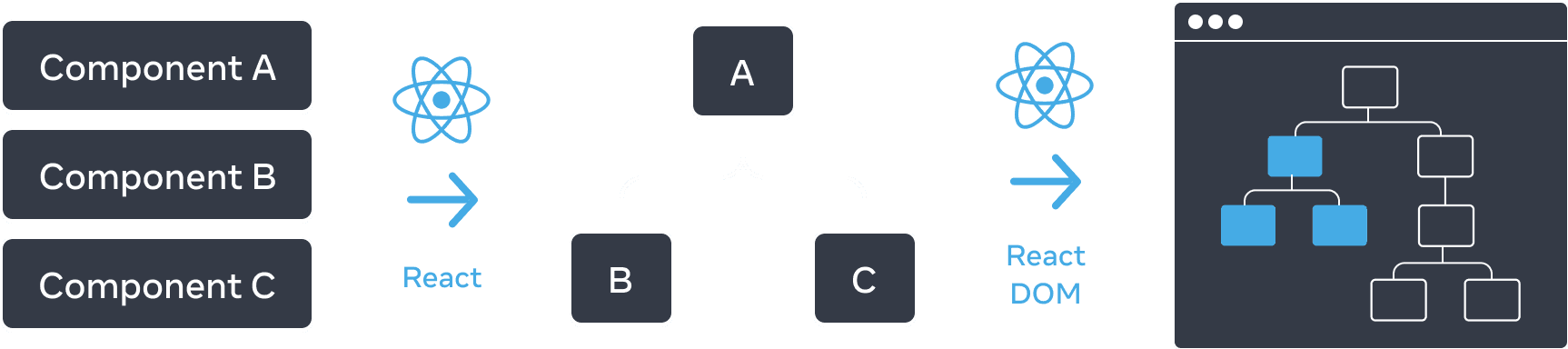
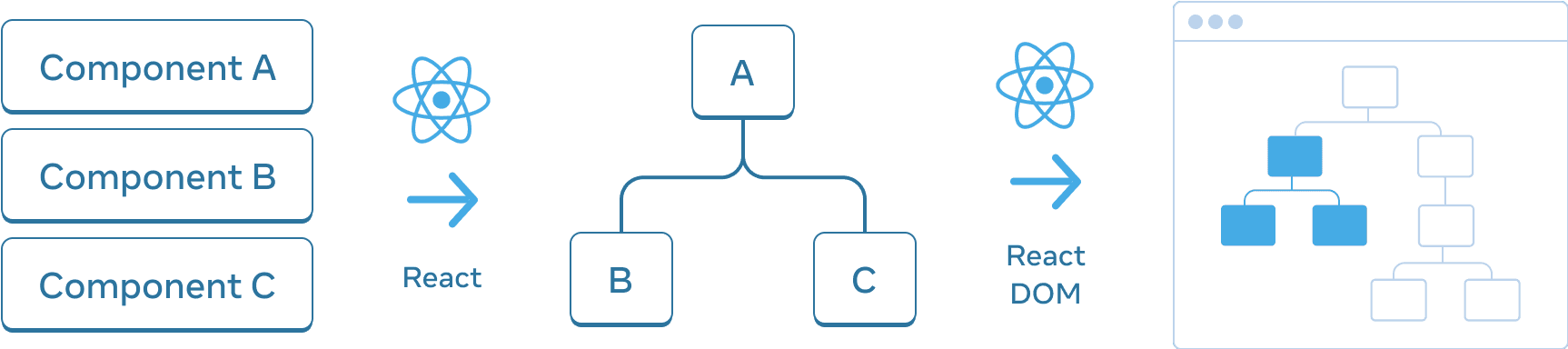
Reactは、コンポーネントからUIツリーを作成します。この例では、UIツリーはDOMにレンダリングするために使用されます。
ブラウザやモバイルプラットフォームと同様に、Reactもツリー構造を使用して、Reactアプリ内のコンポーネント間の関係を管理およびモデル化します。これらのツリーは、データがReactアプリをどのように流れるかを理解し、レンダリングとアプリのサイズを最適化するための便利なツールです。
レンダリングツリー
コンポーネントの主な機能は、他のコンポーネントのコンポーネントを構成できることです。コンポーネントをネストすると、親コンポーネントと子コンポーネントという概念が生まれ、各親コンポーネントは別のコンポーネントの子になる可能性があります。
Reactアプリをレンダリングする場合、この関係を、レンダリングツリーとして知られるツリーでモデル化できます。
インスピレーションを与える名言をレンダリングするReactアプリを次に示します。
import FancyText from './FancyText'; import InspirationGenerator from './InspirationGenerator'; import Copyright from './Copyright'; export default function App() { return ( <> <FancyText title text="Get Inspired App" /> <InspirationGenerator> <Copyright year={2004} /> </InspirationGenerator> </> ); }
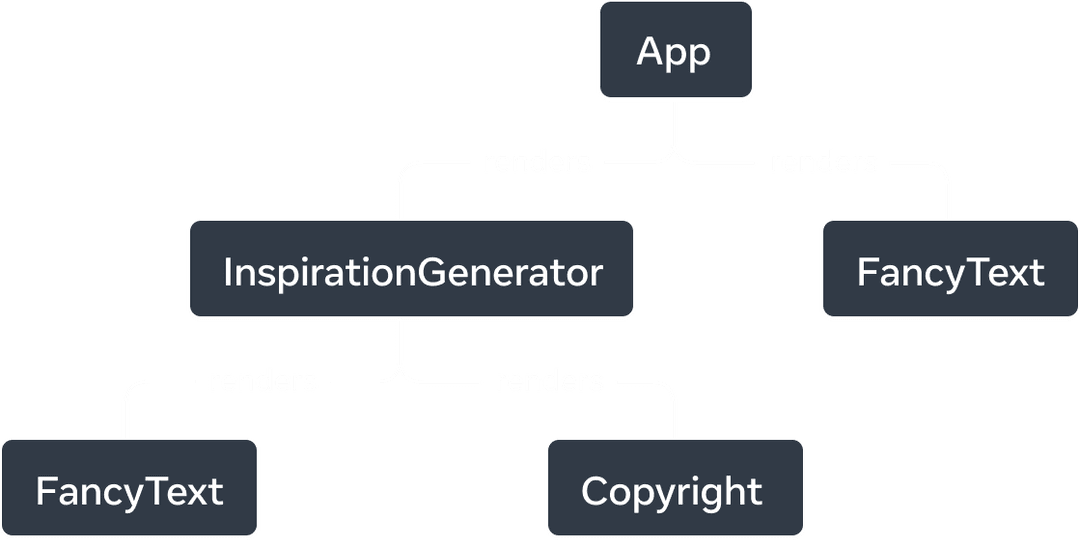
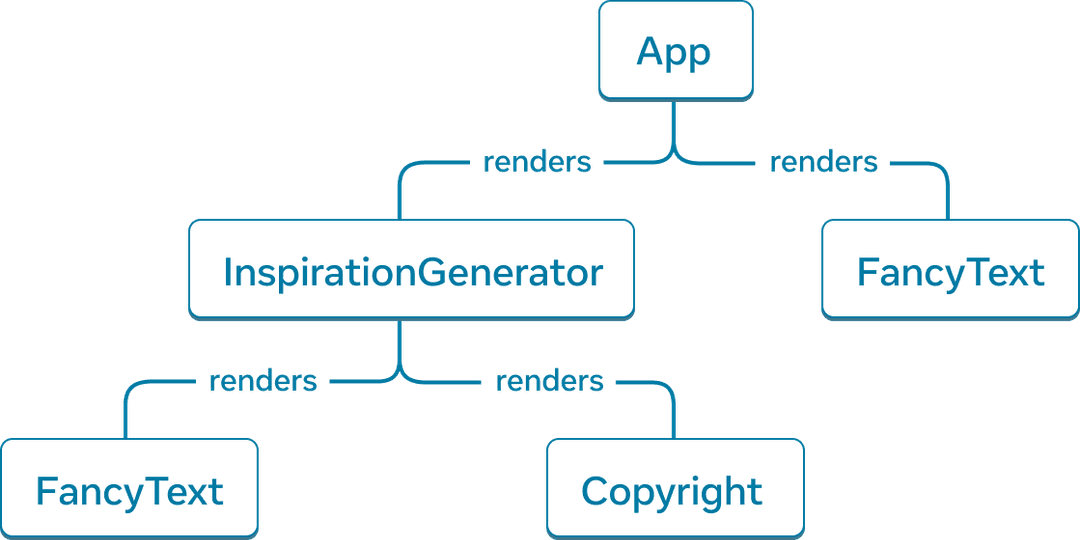
Reactは、レンダリングされたコンポーネントで構成されるUIツリーであるレンダリングツリーを作成します。
サンプルアプリから、上記のレンダリングツリーを構築できます。
ツリーはノードで構成され、各ノードはコンポーネントを表します。App
、FancyText
、Copyright
などを始めとする全てがツリーのノードです。
Reactレンダリングツリーのルートノードは、アプリのルートコンポーネントです。この場合、ルートコンポーネントはApp
であり、Reactが最初にレンダリングするコンポーネントです。ツリー内の各矢印は、親コンポーネントから子コンポーネントを指しています。
深掘り
上記のレンダリングツリーでは、各コンポーネントがレンダリングするHTMLタグについては言及されていません。これは、レンダリングツリーがReactのコンポーネントのみで構成されているためです。
Reactは、UIフレームワークとして、プラットフォームに依存しません。react.devでは、Webにレンダリングする例を紹介しており、UIのプリミティブとしてHTMLマークアップを使用しています。しかし、Reactアプリは、モバイルやデスクトッププラットフォームにレンダリングすることも可能で、その場合はUIViewやFrameworkElementのような異なるUIプリミティブを使用する場合があります。
これらのプラットフォームのUIプリミティブは、Reactの一部ではありません。Reactのレンダリングツリーは、アプリがどのプラットフォームにレンダリングされるかに関わらず、Reactアプリに関する洞察を提供できます。
レンダリングツリーは、Reactアプリケーションの単一のレンダリングパスを表します。条件付きレンダリングを使用すると、親コンポーネントは渡されたデータに応じて異なる子をレンダリングする場合があります。
アプリを更新して、インスピレーションを与える引用句または色のいずれかを条件付きでレンダリングできます。
import FancyText from './FancyText'; import InspirationGenerator from './InspirationGenerator'; import Copyright from './Copyright'; export default function App() { return ( <> <FancyText title text="Get Inspired App" /> <InspirationGenerator> <Copyright year={2004} /> </InspirationGenerator> </> ); }
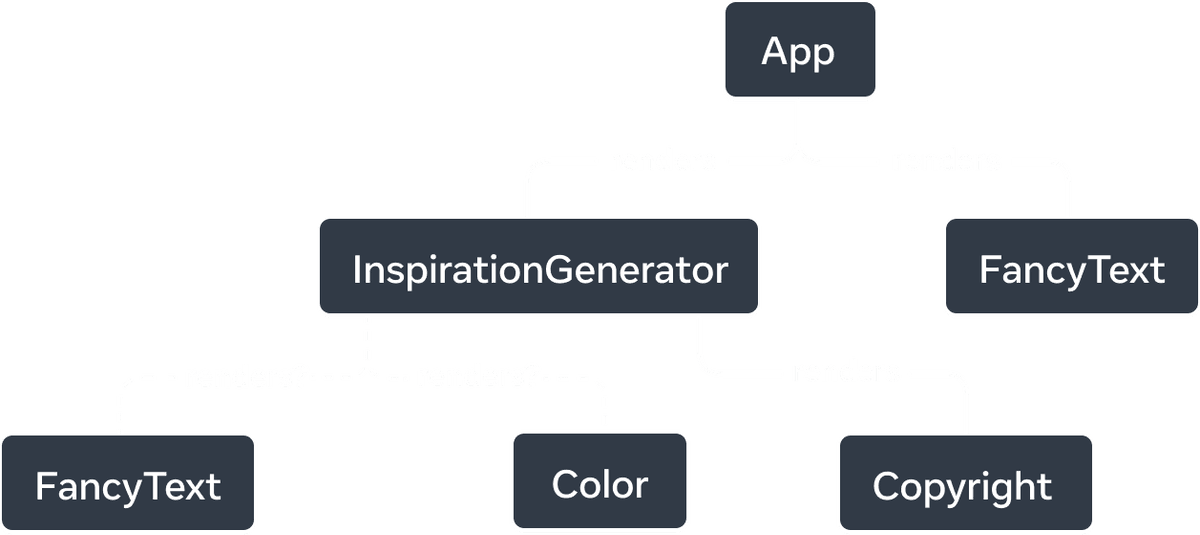
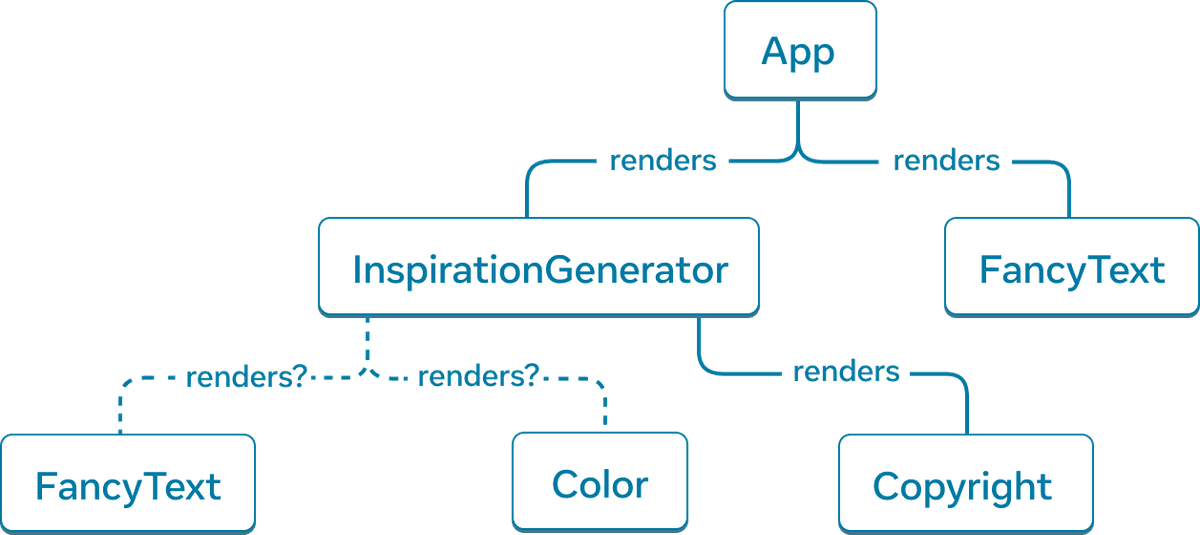
条件付きレンダリングでは、異なるレンダリングにわたって、レンダリングツリーが異なるコンポーネントをレンダリングする場合があります。
この例では、inspiration.type
が何であるかによって、<FancyText>
または<Color>
をレンダリングする場合があります。レンダリングツリーは、各レンダリングパスで異なる場合があります。
レンダリングツリーはレンダリングパス間で異なる場合がありますが、これらのツリーは一般的にReactアプリのトップレベルおよびリーフコンポーネントが何であるかを識別するのに役立ちます。トップレベルコンポーネントは、ルートコンポーネントに最も近く、その下のすべてのコンポーネントのレンダリングパフォーマンスに影響を与え、多くの場合最も複雑な処理を含んでいます。リーフコンポーネントはツリーの下部に近く、子コンポーネントを持たず、頻繁に再レンダリングされます。
これらのカテゴリのコンポーネントを識別することは、アプリのデータフローとパフォーマンスを理解するのに役立ちます。
モジュール依存関係ツリー
ツリーでモデル化できるReactアプリのもう1つの関係は、アプリのモジュール依存関係です。コンポーネントを分割し、ロジックを別々のファイルに分割すると、コンポーネント、関数、または定数をエクスポートできるJSモジュールが作成されます。
モジュール依存関係ツリーの各ノードはモジュールであり、各ブランチはそのモジュール内のimport
ステートメントを表します。
前のInspirationsアプリを使用すると、モジュール依存関係ツリー、または略して依存関係ツリーを構築できます。
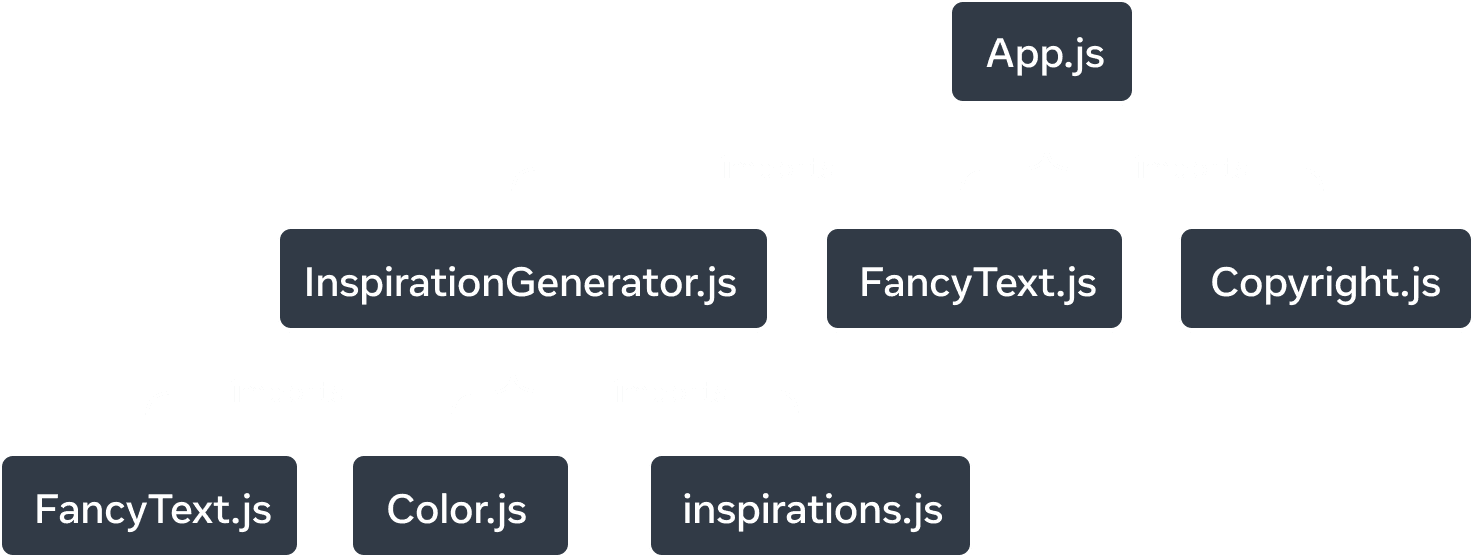
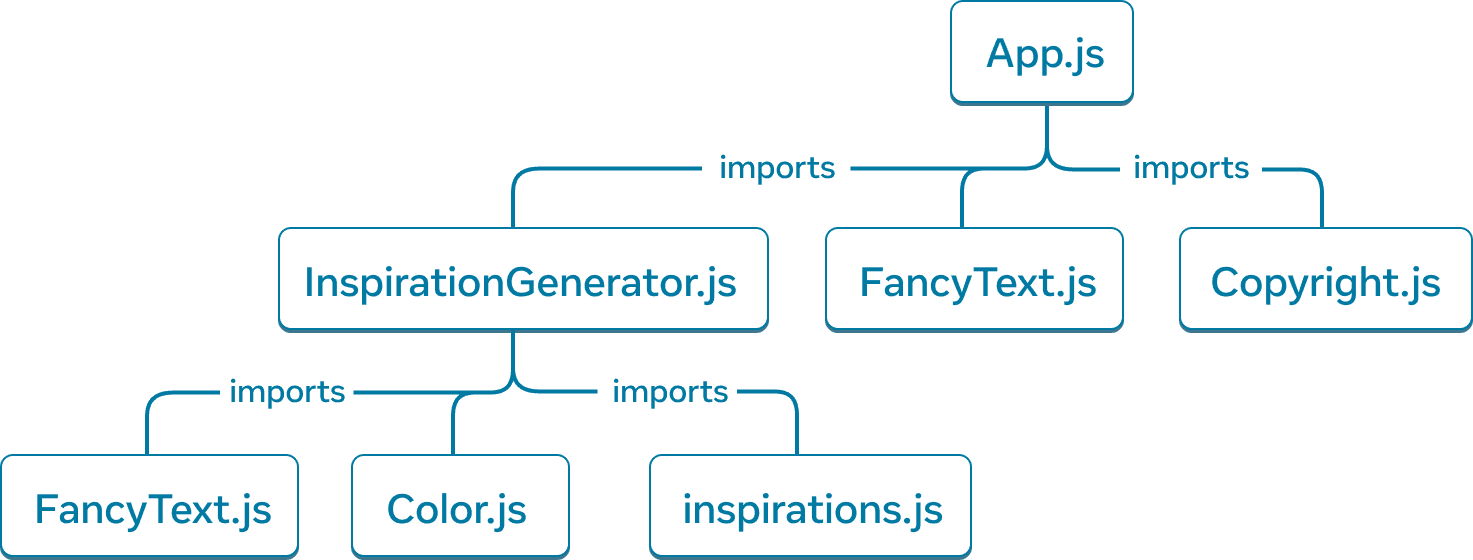
Inspirationsアプリのモジュール依存関係ツリー。
ツリーのルートノードは、ルートモジュールとも呼ばれるエントリポイントファイルです。多くの場合、ルートコンポーネントを含むモジュールです。
同じアプリのレンダリングツリーと比較すると、類似した構造がありますが、いくつかの注目すべき違いがあります。
- ツリーを構成するノードは、コンポーネントではなくモジュールを表します。
inspirations.js
のようなコンポーネント以外のモジュールもこのツリーで表現されます。レンダリングツリーはコンポーネントのみをカプセル化します。Copyright.js
はApp.js
の下に表示されますが、レンダリングツリーでは、コンポーネントであるCopyright
はInspirationGenerator
の子として表示されます。これは、InspirationGenerator
がJSXをchildren propsとして受け入れるため、Copyright
を子コンポーネントとしてレンダリングしますが、モジュールをインポートしないためです。
依存関係ツリーは、Reactアプリを実行するために必要なモジュールを決定するのに役立ちます。本番環境用のReactアプリを構築する場合、通常はクライアントに送信するのに必要なすべてのJavaScriptをバンドルするビルドステップがあります。これを行うツールはバンドラーと呼ばれ、バンドラーは依存関係ツリーを使用して含めるべきモジュールを決定します。
アプリが成長するにつれて、バンドルサイズも大きくなることがよくあります。大きなバンドルサイズは、クライアントがダウンロードして実行するのにコストがかかります。大きなバンドルサイズは、UIが表示されるまでの時間を遅らせる可能性があります。アプリの依存関係ツリーを把握することは、これらの問題をデバッグするのに役立つ場合があります。
まとめ
- ツリーは、エンティティ間の関係を表す一般的な方法です。UIをモデル化するためによく使用されます。
- レンダリングツリーは、単一のレンダリングにおけるReactコンポーネント間のネストされた関係を表します。
- 条件付きレンダリングでは、レンダリングツリーが異なるレンダリング間で変化する可能性があります。異なるprop値を使用すると、コンポーネントは異なる子コンポーネントをレンダリングする可能性があります。
- レンダリングツリーは、トップレベルおよびリーフコンポーネントが何であるかを識別するのに役立ちます。トップレベルコンポーネントは、その下のすべてのコンポーネントのレンダリングパフォーマンスに影響を与え、リーフコンポーネントは頻繁に再レンダリングされることがよくあります。それらを識別することは、レンダリングパフォーマンスを理解し、デバッグするのに役立ちます。
- 依存関係ツリーは、Reactアプリのモジュール依存関係を表します。
- 依存関係ツリーは、アプリを配布するために必要なコードをバンドルするためにビルドツールによって使用されます。
- 依存関係ツリーは、描画までの時間を遅くする大きなバンドルサイズをデバッグしたり、どのコードがバンドルされているかを最適化する機会を見つけるのに役立ちます。